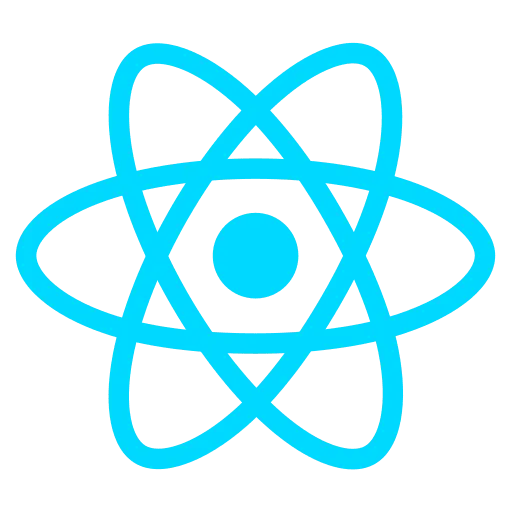
React is a powerful JavaScript library for building user interfaces. It’s popular for its component-based architecture, virtual DOM, and ease of use. However, building truly great React applications requires more than just basic knowledge of the library. Here are 10 best practices you can follow to level up your front-end development skills and write clean, maintainable, and performant React code:
- Component-Based Architecture:
Break down your UI into reusable components, each with its own state, props, and logic. This promotes modularity, makes your code easier to understand and maintain, and allows for independent development and testing of components.
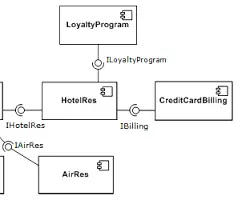
- State Management with Care:
React’s state management can be powerful, but use it cautiously. Consider alternatives like Context API or Redux for complex state management, especially in larger applications.
- Immutable Data and Pure Components:
Use immutable data structures (objects and arrays that can’t be directly modified) and write pure components (functions that always return the same output for the same input). This improves performance and simplifies reasoning about your code.
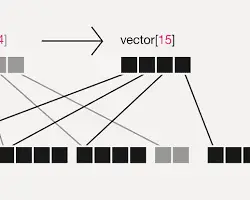
- Avoid Direct DOM Manipulation:
React works best when you interact with the DOM through its virtual DOM abstraction. Avoid directly manipulating the DOM using imperative methods like document.getElementById.
- Keys for Iterating Elements:
When iterating over elements in a list, provide unique keys to help React efficiently update the DOM. This optimizes performance and prevents unnecessary re-renders.
- Conditional Rendering:
Render components or elements conditionally based on state or props. This avoids unnecessary DOM elements and keeps your UI lean and performant.
- Code Splitting and Lazy Loading:
Split your code into smaller chunks and load them only when needed. This improves initial load times and avoids loading unnecessary code.
- Error Boundaries:
Wrap components in error boundaries to handle errors gracefully and prevent cascading failures in your application.
- Performance Optimization:
Pay attention to performance! Use tools like React Profiler and Chrome DevTools to identify bottlenecks and optimize your code for smooth and responsive UI.
- Testing and Documentation:
Write unit and integration tests for your components and document your code clearly. This makes your code more reliable, maintainable, and easier to collaborate on.
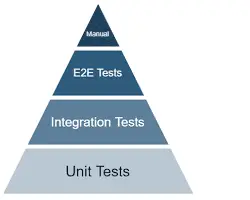
By following these best practices, you can write cleaner, more maintainable, and performant React code. Remember, there’s always more to learn in the ever-evolving world of front-end development. Keep practicing, experimenting, and learning to stay ahead of the curve and build amazing React applications!
These are just some of the best practices for front-end development in React. As with any development methodology, the best approach will depend on the specific project you’re working on. However, by keeping these tips in mind, you can write cleaner, more maintainable code that will help you build better React applications.
I hope this blog post has been helpful. If you have any questions, please feel free to leave a comment below.
Happy coding!